Example
Usage
Users always have two options to upload files from their devices. They can either drag and drop the desired files into the designated area or open their local file system by clicking inside. Two different sizes can be set via a property. We use the smaller, compact version inline, e.g., in forms, whereas the larger version is used as a standalone e.g., in modals.
Anatomy
Dropzone (default)
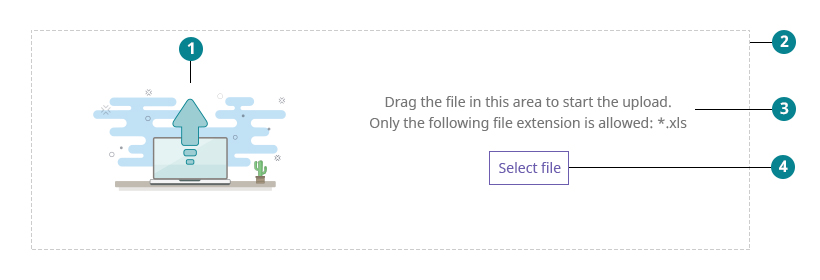
- Image.
https://cdn.wescale.com/wescale/wui/images/cta-feedback/upload-item.svg
- Border. Changes depending on status - initial
2px dashed #ddd
- Hint text (optional). Explanation of file types, allowed size, etc.
- Button. Opens the local file system to select and upload files. The same function is also triggered when clicking anywhere within the dropzone.
Preview file list
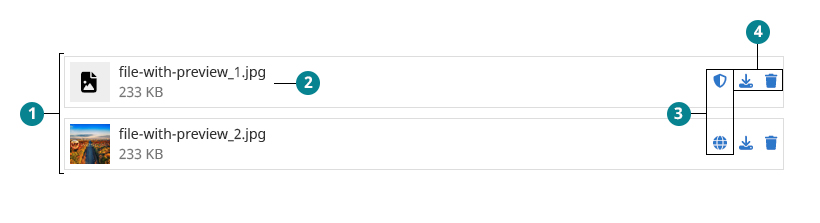
- Preview file list. This list is our default. The items of the preview file list contain essential information about the status of each file. For example, they show whether files are queued, broken, or in progress. We always use the preview item list to make this important information visible to the user, with only a few exceptions. Do not mix list types.
- File information. File name + file size.
- Visibility (optional). This icon is an optional toggle button and shows whether a file is visible internally (
fa-solid fa-shield-halved
) or externally (fa-solid fa-globe
). - Icons. These icons are mandatory.
On (fa-solid fa-download
) the file can be downloaded again.
On (fa-solid fa-trash
) the file can be deleted.
The thumbnails in the file list are generated within the dropzone component during the upload process. However, once the images are uploaded and stored in the database, it is not readily possible to display thumbnails in the file list. In such cases, we simply show the corresponding file type icon instead.
Variants
Dropzone (compact)
Example
The compact variant of the dropzone behaves similarly to the default regarding the states and the file list. It can be integrated well into forms and is often used in conjunction with the crop & resize component.
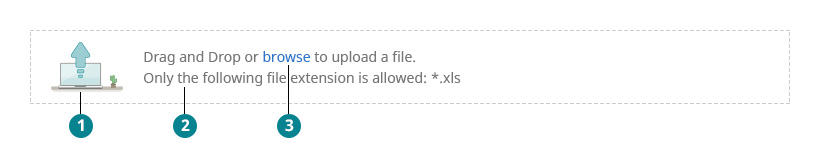
- Image.
https://cdn.wescale.com/wescale/wui/images/cta-feedback/upload-item--sm.svg
- Hint text. Explanation of file types, allowed size, etc.
- Open file system. The
browse link
is a visual anchor for the users to open their file system. Generally, the file system opens whenever the user clicks anywhere within the drop area.
Preview file list (compact)

- Preview file list (compact). It is used especially when we expect many uploads.
Do not mix list types. - File information. The icon (
fa-regular fa-paperclip
) may change and serves as an indicator for different states. - Visibility (optional). This icon is an optional toggle button and shows whether a file is visible internally (
fa-solid fa-shield-halved
) or externally (fa-solid fa-globe
). - Icons. These icons are mandatory.
On (fa-solid fa-download
) the file can be downloaded again.
On (fa-solid fa-trash
) the file can be deleted.
States
Dropzone
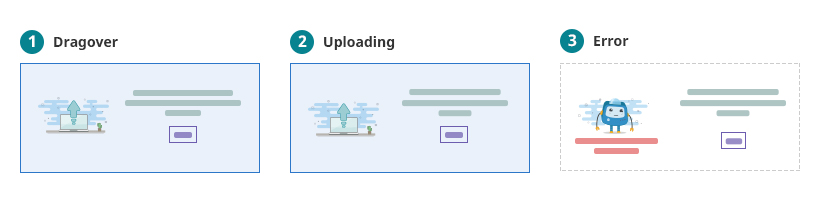
- Dragover. With dragover, the background becomes slightly blue ($color-active-10) and the border becomes $color-active.
- Uploading. When active, the border is $color-active and the background is $color-active-10. The actual progress is displayed for the user within the item preview list.
All options for uploading more items are still available during the "uploading state". - Error. In case of an error, we display this image
https://cdn.wescale.com/wescale/wui/images/system-feedback/unsuccessful-willy-wescale.svg
.
In addition, an error message appears below the image describing the current error. This also applies in the case that only one of several files is erroneous. The upload of new files is still possible.
Preview file list
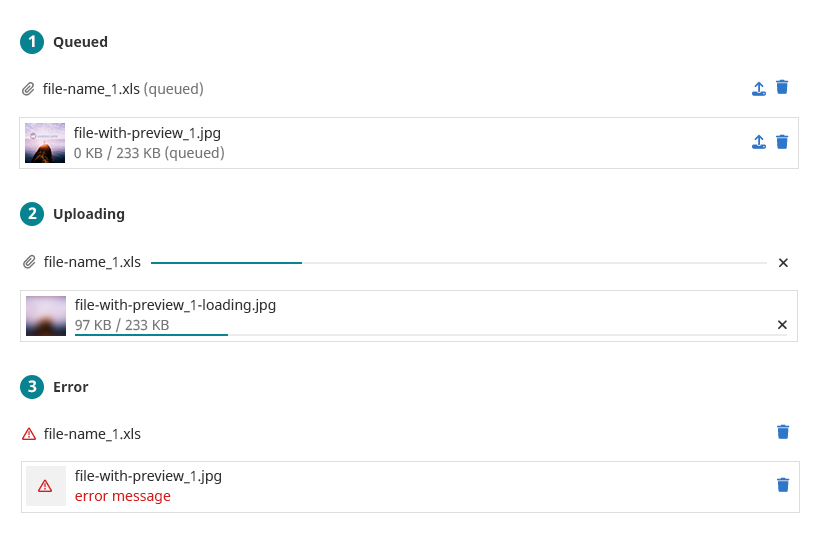
- Queued. We usually skip the queued status and upload the files directly to create a more seamless experience for the user. If the files are staged for technical reasons, there is the possibility to upload all at once via a primary button, or to upload each file itself via the optional icon button
- Uploading. For both types of file lists, there is a progress bar and a "" to cancel the upload. In the default file list there is an additional thumbnail and the display of the progress in KB.
- Error. A broken file is marked within the file list with In the file list compact the error text appears on hover in a tooltip whereas the error text in the default file list is always visible for the user.
WUI decisions 
- Zero-byte file. Yes, there are zero-byte files out there. To prevent technical issues we check each file if it is greater than 0 bytes.
- File size. The maximum size allowed for a file depends very much on the use case. Find a reasonable maximum size in your team. Also, consider the possible sum of file sizes for multiple uploaded files.
- Thumbnails. In the preview file list, we always show a thumbnail image (if available). This applies to all common file formats such as _.pdf, _.doc, *.xls, etc.
- Upload process. Since an upload may take longer, always consider the interaction with other actions on the page. For example, a possible "update-item-button" needs to be disabled during the process of an upload.
- Layout. The file list (regardless of type) is always located below the dropzone.
Description
Component library to create a HTML5-compliant drag'n'drop zone for files.
Some of WUI Dropzone's highlights are:
- Extensive drop zone features
- Image thumbnail previews
- Progress Bars
- Multiple files and synchronous uploads
- Support for large files
- Chunked uploads (single files can be uploaded as chunks in multiple HTTP requests)
- Custom layouts by provided slots
- Browser image resizing (resize the images before you upload them to your server)
- Well tested
Usage
<template>
<wui-dropzone url="/url/for/upload" />
</template>
<!-- wui-dropzone.vue -->
If you want to access file contents you have to use the FileReader API:
<template>
<wui-dropzone url="/url/for/upload" @drop="onDrop" />
</template>
<script>
export default {
methods: {
onDrop(acceptedFiles) {
acceptedFiles.forEach((file) => {
const reader = new FileReader();
reader.onabort = () => console.log("file reading was aborted");
reader.onerror = () => console.log("file reading has failed");
reader.onload = () => {
// Do whatever you want with the file contents
const binaryStr = reader.result;
console.log(binaryStr);
};
reader.readAsArrayBuffer(file);
});
},
},
};
</script>
<!-- wui-dropzone-basic-drop-event.vue -->
Custom Dropzones
You can also create your own drop zone by using the scoped default slot. It provides four getters which just binds the necessary handlers to create a drag 'n' drop zone. Use the getRootProps()
and getRootListeners()
fn's to get the props and events required for drag 'n' drop and use them on any element. For click and keydown behavior, use the getInputProps()
and getInputListeners()
fn's and use the returned props and events on an <input>
.
Furthermore, the functions supports folder drag 'n' drop by default. See file-selector for more info about supported browsers.
The two root getters can be applied to whatever element you want, whereas the two input getters must be applied to an <input>
:
<template>
<wui-dropzone>
<template
#default="{ getRootProps, getRootListeners, getInputProps, getInputListeners }"
>
<div v-bind="getRootProps()" v-on="getRootListeners()">
<input v-bind="getInputProps()" v-on="getInputListeners()" />
</div>
</template>
</wui-dropzone>
</template>
<script>
import { mixinDropzone } from "./helpers/mixin-dropzone";
export default {
mixins: [mixinDropzone],
};
</script>
<!-- wui-dropzone-custom.vue -->
Note that whatever other props or events you want to add to the element where the props from getRootProps()
or getRootListeners()
are set, you should always pass them through that function rather than applying them on the element itself. This is in order to avoid your props and events being overridden (or overriding the props and events returned by getRootProps()
/ getRootListeners()
):
<div
v-bind="getRootProps({
class: 'my-dropzone',
role: 'button',
'aria-label': 'drag and drop area',
...
})"
v-on="getRootListeners({ click: event => console.log(event) })"
/>
In the example above, the provided click
handler will be invoked before the internal one, therefore, internal callbacks can be prevented by simply using stopPropagation.
Important: if you omit rendering an <input>
and/or binding the props and events from getInputProps()
and getInputListeners()
, opening a file dialog will not be possible.
Caveats
File Dialog Cancel Callback Event
The file-dialog-cancel
event is unstable in most browsers, meaning, there's a good chance of it being triggered even though you have selected files.
We rely on using a timeout of 300ms after the window is focused (the window onfocus event is triggered when the file select dialog is closed) to check if any files were selected and trigger file-dialog-cancel
if none were selected.
As one can imagine, this doesn't really work if there's a lot of files or large files as by the time we trigger the check, the browser is still processing the files and no onchange events are triggered yet on the input.
Fortunately, there's the File System Access API, which is currently a working draft and some browsers support it see browser compatibility), that provides a reliable way to prompt the user for file selection and capture cancellation.
Also keep in mind that the FS access API can only be used in secure contexts.
NOTE You can disable using the FS access API by setting the useFsAccessApi
prop to false
.
Supported Browsers
We use browserslist config to state the browser support for this lib, so check it out on browserslist.dev.
Need image editing?
To create a modern image editing experience you can use Pintura Image Editor. Pintura supports crop aspect ratios, resizing, rotating, cropping, annotating, filtering, and much more.
Compact Mode
The drop zone can be rendered in a collapsed layout if the compact
property is set to true
. The file list can also be rendered in a collapsed layout if compactFileList
is set to true
.
<template>
<wui-dropzone compact compact-file-list />
</template>
<!-- wui-dropzone-compact.vue -->
Event Propagation
If you'd like to selectively turn off the default dropzone behavior for click
, use the noClick
property:
<template>
<wui-dropzone url="/url/for/upload" no-click />
</template>
<!-- wui-dropzone-no-click.vue -->
If you'd like to selectively turn off the default dropzone behavior for keyDown
, focus
and blur
, use the noKeyboard
property:
<template>
<wui-dropzone url="/url/for/upload" no-keyboard>
<template #info">
<p class="text-center">Dropzone without keyboard events</p>
<em class="text-center">(SPACE/ENTER and focus events are disabled)</em>
</template>
</wui-dropzone>
</template>
<!-- wui-dropzone-no-keyboard.vue -->
If you'd like to selectively turn off the default dropzone behavior for drag events, use the noDrag
property:
<template>
<wui-dropzone url="/url/for/upload" no-drag>
<template #info">
<p class="text-center">Dropzone with no drag events</p>
<em class="text-center">(Drag 'n' drop is disabled)</em>
</template>
</wui-dropzone>
</template>
<!-- wui-dropzone-no-drag.vue -->
Keep in mind that if you provide your own callback handlers as well and use event.stopPropagation()
, it will prevent the default dropzone behavior:
<template>
<wui-dropzone url="/url/for/upload" @drop="onDrop">
<template #info">
<p class="text-center">Dropzone with no drag events</p>
<em class="text-center">(Drag 'n' drop is disabled)</em>
</template>
</wui-dropzone>
</template>
<script>
export default {
methods: {
onDrop(acceptedFiles, rejectedFiles, event) {
event.stopPropagation();
},
},
};
</script>
<!-- wui-dropzone-events-stop-propagation.vue -->
Accepting specific file types
By providing accept
prop you can make the dropzone accept specific file types and reject the others.
The value must be an object with a common MIME type as keys and an array of file extensions as values (similar to showOpenFilePicker's types accept option).
accept: {
'image/png': ['.png'],
'text/html': ['.html', '.htm'],
}
For more information see https://developer.mozilla.org/en-US/docs/Web/HTML/Element/Input.
<template>
<wui-dropzone
:accept="{
'image/jpeg': [],
'image/png': []
}"
>
<template #info">
<p class="text-center">Drag 'n' drop some files here, or click to select files</p>
<em class="text-center">(Only *.jpeg and *.png images will be accepted)</em>
</template>
</wui-dropzone>
</template>
<!-- wui-dropzone-accept.vue -->
Browser limitations
Because of HTML5 File API differences across different browsers during the drag, Dropzone might not be able to detect whether the files are accepted or rejected in Safari nor IE.
Furthermore, at this moment it's not possible to read file names (and thus, file extensions) during the drag operation. For that reason, if you want to react on different file types during the drag operation, you have to use mime types and not extensions! For example, the following example won't work even in Chrome:
<template>
<wui-dropzone :accept="{ 'image/jpeg': ['.jpeg', '.png'] }">
<template #info={ isDragActive, isDragAccept, isDragReject, }">
<p v-if="isDragAccept" class="text-center">All files will be accepted</p>
<p v-if="isDragReject" class="text-center">Some files will be rejected</p>
<p v-if="!isDragActive" class="text-center">Drop some files here ...</p>
</template>
</wui-dropzone>
</template>
<!-- wui-dropzone-accept-not-working.vue -->
but this one will:
<template>
<wui-dropzone :accept="{ 'image/*': ['.jpeg', '.png'] }">
<template #info={ isDragActive, isDragAccept, isDragReject, }">
<p v-if="isDragAccept" class="text-center">All files will be accepted</p>
<p v-if="isDragReject" class="text-center">Some files will be rejected</p>
<p v-if="!isDragActive" class="text-center">Drop some files here ...</p>
</template>
</wui-dropzone>
</template>
<!-- wui-dropzone-accept-working.vue -->
Notes
Mime type determination is not reliable across platforms. CSV files, for example, are reported as text/plain under macOS but as application/vnd.ms-excel under Windows. In some cases there might not be a mime type set at all.
Accepting specific number of files
By providing maxFiles
prop you can limit how many files the dropzone accepts.
Note that this prop is enabled when the multiple
prop is enabled. The default value for this prop is 0
, which means there's no limitation to how many files are accepted.
<template>
<wui-dropzone :max-files="2" />
</template>
<!-- wui-dropzone-max-files.vue -->
Custom validation
By providing validator
prop you can specify custom validation for files.
The value must be a function that accepts File object and returns null if file should be accepted or error object/array of error objects if file should be rejected.
<template>
<wui-dropzone :validator="nameLengthValidator" />
</template>
<script>
export default {
data() {
return {
maxLength: 20,
};
},
methods: {
nameLengthValidator(file) {
if (file.file.name.length > this.maxLength) {
return {
code: "name-too-large",
message: `Name is larger than ${this.maxLength} characters`,
};
}
return null;
},
},
};
</script>
<!-- wui-dropzone-validator.vue -->
Opening File Dialog Programmatically
You can programmatically invoke the default OS file prompt; just use the open
instance method provided by most of the scoped slots (e. h. #info).
Note that for security reasons most browsers require popups and dialogues to originate from a direct user interaction (i.e. click).
If you are calling open()
asynchronously, there’s a good chance it’s going to be blocked by the browser. So if you are calling open()
asynchronously, be sure there is no more than 1000ms delay between user interaction and open()
call.
Due to the lack of official docs on this (at least we haven’t found any. If you know one, please let us know), there is no guarantee that allowed delay duration will not be changed in later browser versions. Since implementations may differ between different browsers, avoid calling open asynchronously if possible.
<template>
<!-- // Disable click and keydown behavior -->
<wui-dropzone no-click no-keyboard>
<template #info={ open }">
<p class="text-center">Drag 'n' drop some files here ...</p>
<wui-button @click="open">Open File Dialog</wui-button>
</template>
</wui-dropzone>
</template>
<!-- wui-dropzone-open-file-dialog-programmatically.vue -->
Upload Queue Manually
When a file is added to the drop zone, its status is set to queued
(if files pass the internal validation check), meaning the file is now in the queue.
If you have the autoProcessQueue
option set to true
, the queue will be processed immediately after a file is dropped or an upload is finished by calling .processQueue()
which checks how many files are currently being uploaded.
If you set autoProcessQueue
to false
then .processQueue()
will never be called implicitly. This means that if you want to upload all the files currently in the queue, you have to invoke .processQueue()
yourself.
<template>
<wui-dropzone :auto-process-queue="false">
<template #info="{ queuedFiles, processQueue }">
<p class="text-center">Drag 'n' drop some files here ...</p>
<wui-button v-if="queuedFiles.length" @click="processQueue"
>Upload files</wui-button
>
</template>
</wui-dropzone>
</template>
<!-- wui-dropzone-process-queue-manually.vue -->
Extending Dropzone
The component accepts a getFilesFromEvent
prop that enhances the handling of dropped file system objects and allows more flexible use of them e.g. passing a function that accepts drop event of a folder and resolves it to an array of files adds plug-in functionality of folders drag-and-drop.
Though, note that the provided getFilesFromEvent
fn must return a Promise
with a list of File
objects (or DataTransferItem
of {kind: 'file'}
when data cannot be accessed). Otherwise, the results will be discarded and none of the drag events callbacks will be invoked.
In case you need to extend the File
with some additional properties, you should use Object.defineProperty()
so that the result will still pass through our filter:
<template>
<wui-dropzone :get-files-from-event="myCustomFileGetter" />
</template>
<script>
export default {
methods: {
myCustomFileGetter(event) {
const files = [];
const fileList = event.dataTransfer
? event.dataTransfer.files
: event.target.files;
for (var i = 0; i < fileList.length; i++) {
const file = fileList.item(i);
Object.defineProperty(file, "myProp", {
value: true,
});
files.push(file);
}
return files;
},
},
};
</script>
<!-- wui-dropzone-get-files-from-event.vue -->
Custom Layouts
There are scoped slots for almost each part of the drop zone to render custom content.
default
slot
The default
scoped slot replaces the entire drop zone with custom content, but not the preview file list. Make sure you use the four getter functions from the slot scope for your drop zone root element (getRootProps()
, getRootListeners()
) and for the input form element (getInputProps()
, getInputListeners()
) to provide the drop zone functionality.
<template>
<wui-dropzone>
<template
#default="{
getRootProps,
getRootListeners,
getInputProps,
getInputListeners,
open,
}"
>
<div
v-bind="getRootProps({ class: 'my-dropzone-root' })"
v-on="getRootListeners()"
>
<input v-bind="getInputProps()" v-on="getInputListeners()" />
<p class="text-center">Drag 'n' drop some files here ...</p>
<p class="text-center">or</p>
<wui-button @click="open">Select files</wui-button>
</div>
</template>
</wui-dropzone>
</template>
<!-- wui-dropzone-default-scoped-slot.vue -->
label
slot
The label
scoped slot replaces almost all of the drop zone with custom content, except for the drop zone root element and its functionality (mostly drag 'n' drop) and the preview file list, which remain. Since it also replaces the file element like the default slot, you have to create this element yourself and use the two getter functions (getInputProps()
and getInputListeners()
).
<template>
<wui-dropzone>
<template
#label="{
getInputProps,
getInputListeners,
open,
}"
>
<label :id="dz-label" for="file-input" class="my-dropzone-label">
<input
v-bind="getInputProps({ id: 'file-input' })"
v-on="getInputListeners()"
/>
<p class="text-center">Drag 'n' drop some files here ...</p>
<p class="text-center">or</p>
<wui-button @click="open">Select files</wui-button>
</label>
</template>
</wui-dropzone>
</template>
<!-- wui-dropzone-label-scoped-slot.vue -->
image
slot
The image
scoped slot replaces the drop zone wescale image with custom content.
busy
slot
The busy
scoped slot replaces the drop zone's default busy
state with your own custom content, e. g. a custom spinner. The busy scoped slot is only rendered if disablePreviews
is true
and multiple
is false
and a file is uploading.
<template>
<wui-dropzone>
<template
#busy="{
file
fileSize,
uploadedFileSize,
cancelUpload,
}"
>
<div class="w-full">
<span>{ file.file.name } </span>
<div
class="display--flex align-items-center justify-content-between"
:style="compact && { order: 6 }"
>
<p class="m-0">
<span class="text-muted">
<span
>{ uploadedFileSize.value } { uploadedFileSize.unit } / </span
>{ fileSize.value } { fileSize.unit }</span
>
</p>
<wui-button
outline
variant="link"
class="wui-color--default"
:title="t('dropzone.button.cancelUpload')"
@click.stop="cancelUpload"
><wui-fa-icon icon="xmark"
/></wui-button>
</div>
<wui-progress
:value="file.upload.progress"
class="flex-fill"
height="2px"
/>
</div>
</template>
</wui-dropzone>
</template>
<!-- wui-dropzone-busy-scoped-slot.vue -->
info-container
slot
The info-container
slot replaces the complete content on the right side of the drop zone, as the text and the "Select files" button.
info
slot
The info
slot replaces the content on the right side of the drop zone, except for the "Select files" button, which remains.
info-message
slot
The info-message
slot replaces only the first text (dropzone.message
) on the right side of the drop zone.
info-message-allowed-extensions
slot
The info-message-allowed-extensions
slot replaces only the text for allowed file extensions (dropzone.messageAllowedExtensions
) on the right side of the drop zone.
file-actions
slot
Use the file-actions
scoped slot to add one or more action icon buttons to every file preview.
<template>
<wui-dropzone>
<template #file-actions="{ id, status }">
<wui-button
v-if="status === 'success'"
variant="link"
title="Change visibility"
@click="handleChangeVisibility(id)"
>
<wui-fa-icon
:icon="getIsFileVisibileById(id) ? 'globe' : 'shield-halved'"
/>
</wui-button>
</template>
</wui-dropzone>
</template>
<script>
export default {
data() {
return {
internalFileIds: [],
};
},
methods: {
getIsFileVisibileById(id) {
return Boolean(this.internalFileIds.every((_id) => _id !== id));
},
deleteInternalFileStateById(id) {
const index = this.internalFileIds.findIndex((_id) => _id === id);
if (index > -1) this.internalFileIds.splice(index, 1);
},
handleChangeVisibility(id) {
if (this.getIsFileVisibileById(id)) {
this.internalFileIds.push(id);
return;
}
this.deleteInternalFileStateById(id);
},
},
};
</script>
<!-- wui-dropzone-file-actions-scoped-slot.vue -->
File list helper components
WuiVue provides additional helper child components for previewing files:
wui-dropzone-file-list
(aliaswui-file-list
)wui-dropzone-file-list-item
(aliaswui-file-list-item
)
These components serve the purpose of displaying a custom preview of dropped or selected files elsewhere in your app.
file-list
slot
With the file-list
scoped slot you can render a custom preview of the files. Optionally, you can use the helper components mentioned above to create a consistent layout.
<template>
<wui-dropzone>
<template #file-list="{ files, remove, cancelUpload }">
<p>Add some custom text or any other content here ...</p>
<!-- You can use our helper components or your own layout -->
<wui-dropzone-file-list v-if="files.length">
<wui-dropzone-file-list-item
v-for="file of files"
:id="file.upload.uuid"
:key="file.upload.uuid"
:errors="file.errors"
:file-name="file.file.name"
:image-url="file.thumbnailDataURL"
:bytes-sent="file.upload.bytesSent"
:progress="file.upload.progress"
:size="file.file.size"
:status="file.status"
tag="li"
:type="file.file.type"
:add-download-link
@download="handleDownload"
@remove="remove"
@cancel-upload="cancelUpload"
/>
</wui-dropzone-file-list>
</template>
</wui-dropzone>
</template>
<script>
export default {
methods: {
// `id` is the unique file.upload.uuid
handleDownload(id) {
// do something
},
},
};
</script>
<!-- wui-dropzone-file-list-scoped-slot.vue -->
Component reference
<wui-dropzone>
Properties
Property | Type | Default | Description |
---|---|---|---|
accept | Object | {} | Set accepted file types. Checkout https://developer.mozilla.org/en-US/docs/Web/API/window/showOpenFilePicker types option for more information. Keep in mind that mime type determination is not reliable across platforms. CSV files, for example, are reported as text/plain under macOS but as application/vnd.ms-excel under Windows. In some cases there might not be a mime type set at all |
addDownloadLinks | Boolean | false | Whether or not a download action button will be displayed in the preview file list |
autoFocus | Boolean | false | Set to true to focus the root element on render |
autoProcessQueue | Boolean | true | If false , files will be added to the queue but the queue will not be processed automatically. This can be useful if you need some additional user input before sending files (or if you want want all files sent at once). If you're ready to send the file simply call $refs.myDropzone.processQueue() . See docs section for more information. |
autoQueue | Boolean | true | If false , files added to the dropzone will not be queued by default. You'll have to call $refs.myDropzone.enqueueFile(file) manually. |
binaryBody | Boolean | false | Sends the file as binary blob in body instead of form data. If this is set, the params option will be ignored. It's an error to set this to true along with uploadMultiple since multiple files cannot be in a single binary body. |
chunkSize | Number | 2097152 | If chunking is true , then this defines the chunk size in bytes. |
chunking | Boolean | false | Whether you want files to be uploaded in chunks to your server. This can't be used in combination with uploadMultiple . See docs for the callback to finalise an upload. |
compact | Boolean | false | If true, renders dropzone in compact layout |
compactFileList | Boolean | false | Whether or not the preview file list should be rendered in compact mode |
createImageThumbnails | Boolean | true | Whether thumbnails for images should be generated |
defaultHeaders | Boolean | true | Should the default headers be set or not? Accept: application/json <- for requesting json response, Cache-Control: no-cache <- request shouldnt be cached, X-Requested-With: XMLHttpRequest <- we sent the request via XMLHttpRequest |
disablePreviews | Boolean | false | Set this to true if you don't want previews to be shown. |
disabled | Boolean | false | Enable/disable the dropzone |
forceChunking | Boolean | false | If chunking is enabled, this defines whether every file should be chunked, even if the file size is below chunkSize. This means, that the additional chunk form data will be submitted and the chunks-uploaded event will be emitted. |
getFilesFromEvent | Function | undefined | Use this to provide a custom file aggregator. Arguments event : (DragEvent - Event ) — A drag event or input change event (if files were selected via the file dialog) |
headers | Object | {} | An optional object to send additional headers to the server. Eg: { "My-Awesome-Header": "header value" } |
id | String | 'dropzone' | Used to set the id attribute on the rendered content, and used as the base to generate any additional element IDs as needed |
maxFiles | Number | 0 | Maximum accepted number of files The default value is 0 which means there is no limitation to how many files are accepted. |
maxSize | Number | Infinity | Maximum file size (in bytes) |
maxSizeTotal | Number | Infinity | Maximum total size of all files (in bytes) |
maxThumbnailFilesize | Number | 10 | In MB. When the filename exceeds this limit, the thumbnail will not be generated. |
method | String , Function | 'POST' | Can be changed to "put" if necessary. You can also provide a function that will be called with files and must return the method. |
minSize | Number | 0 | Minimum file size (in bytes) |
multiple | Boolean | true | Allow drag 'n' drop (or selection from the file dialog) of multiple files |
noClick | Boolean | false | If true , disables click to open the native file selection dialog |
noDrag | Boolean | false | If true , disables drag 'n' drop |
noDragEventsBubbling | Boolean | false | If true , stops drag event propagation to parents |
noKeyboard | Boolean | false | If true , disables SPACE/ENTER to open the native file selection dialog. Note that it also stops tracking the focus state. |
noRemoveLinks | Boolean | false | If true , will not render the remove action button in the preview file list |
parallelChunkUploads | Boolean | false | If true , the individual chunks of a file are being uploaded simultaneously. |
parallelUploads | Number | 2 | How many file uploads to process in parallel (See docs for more info) |
paramName | String | 'file' | The name of the file param that gets transferred. NOTE: If you have the option uploadMultiple set to true, then wui-dropzone will append [] to the name. |
params | Object , Function | undefined | Can be an object of additional parameters to transfer to the server, or a Function that gets invoked with the files , xhr and, if it's a chunked upload, chunk arguments. In case of a function, this needs to return a map. The default implementation does nothing for normal uploads, but adds relevant information for chunked uploads. This is the same as adding hidden input fields in the form element. |
preventDropOnDocument | Boolean | true | If false, allow dropped items to take over the current browser window |
renameFile | Function | null | A function that is invoked before the file is uploaded to the server and renames the file. This function gets the File as argument and can use the file.name . The actual name of the file that gets used during the upload can be accessed through file.upload.filename . |
resize | Function | undefined | Gets called to calculate the thumbnail dimensions. It gets file , width and height (both may be null ) as parameters and must return an object containing: srcWidth & srcHeight (required), trgWidth & trgHeight (required), srcX & srcY (optional, default 0 ), trgX & trgY (optional, default 0 ). Those values are going to be used by ctx.drawImage() . |
resizeHeight | Number | null | See resizeWidth |
resizeMethod | String | 'contain' | How the images should be scaled down in case both, resizeWidth and resizeHeight are provided. Can be either contain or crop . |
resizeMimeType | String | null | The mime type of the resized image (before it gets uploaded to the server). If null the original mime type will be used. To force jpeg, for example, use image/jpeg . See resizeWidth for more information. |
resizeQuality | Number | 0.8 | The quality of the resized images. See resizeWidth . |
resizeWidth | Number | null | If set, images will be resized to these dimensions before being uploaded. If only one, resizeWidth or resizeHeight is provided, the original aspect ratio of the file will be preserved. The transformFile prop function uses these options, so if the transformFile function is overridden, these options don't do anything. |
retryChunks | Boolean | false | Whether a chunk should be retried if it fails. |
retryChunksLimit | Number | 3 | If retryChunks is true , how many times should it be retried. |
thumbnailHeight | Number | 120 | The same as thumbnailWidth . If both are null, images will not be resized. |
thumbnailMethod | String | 'crop' | How the images should be scaled down in case both, thumbnailWidth and thumbnailHeight are provided. Can be either contain or crop . |
thumbnailWidth | Number | 120 | If null , the ratio of the image will be used to calculate it. |
timeout | Number | null | The timeout for the XHR requests in milliseconds. If set to null or 0 , no timeout is going to be set. |
transformFile | Function | undefined | Can be used to transform the file (for example, resize an image if necessary). The default implementation uses resizeWidth and resizeHeight (if provided) and resizes images according to those dimensions. Gets the file as the first parameter, and a done() function as the second, that needs to be invoked with the file when the transformation is done. |
translations | Object | The translation object to pass custom texts | |
uploadMultiple | Boolean | false | Whether to send multiple files in one request. If this it set to true , then the fallback file input element will have the multiple attribute as well. This option will also trigger additional events (like processingmultiple ). See docs for more information. |
url | String , Function | '' | Has to be specified on elements other than form (or when the form doesn't have an action attribute). You can also provide a function that will be called with files and must return the url. |
useFsAccessApi | Boolean | true | Set to true to use the https://developer.mozilla.org/en-US/docs/Web/API/File_System_Access_API to open the file picker instead of using an <input type="file"> click event. |
validator | Function | null | Custom validation function. It must return null if there's no errors. Arguments: file : File - returns FileError - Array.<FileError> - null ) |
withCredentials | Boolean | false | Will be set on the XHRequest. |
Slots
Name | Scoped | Description |
---|---|---|
default | Yes, see package.json | Content to place in the dropzone. Will remove the drop zone's behaviour. You need to bind the getter functions getRootProps() , getRootListeners() , getInputProps() and getInputListeners() to your drop zone wrapper and input element |
label | Yes, see package.json | Content to place in the drop zone's label. Will remove the drop zone's built-in input element. You need to bind the getter functions getInputProps() and getInputListeners() to your own drop zone's input element |
image | Yes, see package.json | Content to replace the drop zone's image on the left side (only available if compact is false ) |
info-container | Yes, see package.json | Content to replace the drop zone's content on the right side (only available if (disablePreviews && !multiple && isUploading) is false ) |
info | Yes, see package.json | Content to replace the drop zone's content on the right side, except the 'Select files' button |
info-message | Yes, see package.json | Content to replace the drop zone's initial text on the right side, except the 'allowed extensions' text |
info-message-allowed-extensions | Yes, see package.json | Content to replace the drop zone's 'allowed extensions' text on the right side |
file-list | Yes, see package.json | Content to replace the file preview list with. You can use the helper components wui-dropzone-file-list and wui-dropzone-file-list-item to create your own file preview |
busy | Yes, see package.json | Content for the busy state when a file is being processed. Only available if multiple is false , disablePreviews is true and the file state is uploading |
file-actions | Yes, see package.json | Content to place next to the download and remove icon buttons for each file preview item |
Events
Event | Arguments | Description |
---|---|---|
download | file - Object - File object | Emitted when user clicks on a download button |
complete | file - Object - File object | Emitted when file processing and uploading are finished, when an upload got canceled or an error occured during the processing. |
completemultiple | files - Array[file] - An array with file objects | Only emitted when uploadMultiple is true and file processing and uploading are finished, when an upload got canceled or an error occured during the processing. |
queuecomplete | Emitted when file processing and uploading (if validation was successfull) of all queued files have been finished | |
file-added | file - Object - File object | Emitted when one or more files have been added by the file picker or by dropping |
dragenter | dragenter - DragEvent - The native dragenter event | Emitted when the dragged files enter the drop zone's drop target |
dragover | dragover - DragEvent - The native dragover event | Emitted when files are being dragged over the drop zone's drop target (every few hundred milliseconds) |
dragleave | dragleave - DragEvent - The native dragleave event | Emitted when the dragged files leave the drop zone's drop target |
drop | acceptedFiles - Array[File] - Array of all accepted filesrejectedFiles - Array[File] - Array of all rejected filesdrop - DragEvent - The native drop event | Emitted when files have been dropped by the user |
drop-accepted | acceptedFiles - Array[File] - Array of all accepted filesdrop - DragEvent - The native drop event | Emitted after files have been dropped by the user and have been passed the validation |
drop-rejected | rejectedFiles - Array[File] - Array of all rejected filesdrop - DragEvent - The native drop event | Emitted after files have been dropped by the user and have not been passed the validation |
click | wvEvent - WvEvent - WvEvent object | Emitted when the user clicked in the drop zone and the file picker is about to be shown. Cancelable. Call wvEvent.preventDefault() to cancel click |
file-dialog-open | Emitted when the file picker is about to be show | |
file-dialog-cancel | DOMException - DOMException Event - Native DOMException event object | Emitted either when the file picker is canceled by the user or the window gets the focus |
thumbnail | dataUrl - String - The base64 thumbnail urlfile - Object - File object | Emitted when thumbnail processing has been finished |
processing | file - Object - File object | Emitted when upload process of the file is about to start |
processingmultiple | files - Array - Array of file objects | Emitted when uploadMultiple is true and the upload process of the files is about to start |
sending | file - Object - File objectxhr - Object - The XMLHttpRequest objectformData - Object - FormData object (only emitted when binaryBody is false ) | Emitted when the XMLHttpRequest starts sending the file to the server |
sendingmultiple | files - Array - Array of file objectsxhr - Object - The XMLHttpRequest objectformData - Object - FormData object (only emitted when binaryBody is false ) | Emitted when uploadMultiple is true and the XMLHttpRequest starts sending the files to the server |
uploadprogress | file - Object - Object of the file currently uploadingprogress - Number - The current progress in percentbytesSent - Number - The number of bytes which already have been sent | Emitted multiple times during the file upload by the XMLHttpRequest progress event until the upload has been finished |
totaluploadprogress | totalUploadProgress - Number - The current total progress of all files in percenttotalBytes - Number - The total number of bytes of all filestotalBytesSent - Number - The total number of bytes of all files which already have been sent | Emitted multiple times during the files upload by the XMLHttpRequest progress event until all file uploads have been finished |
chunks-uploaded | file - Object - Object of the file currently uploadingresponse - String - The XMLHttpRequest responseText | Emitted when chunking is true and all file chunks have been uploaded |
file-error | file - Object - File objectmessages - Array - An array of error message objects [ { code: String, message: String } ] xhr - Object - The XMLHttpRequest object | Emitted when the added file didn't pass the validation or an XMLHttpRequest error occured during the file upload |
file-errormultiple | files - Array - Array of file objectsmessages - Array - An array of error message objects [ { code: String, message: String } ] xhr - Object - The XMLHttpRequest object | Emitted when uploadMultiple is true and the added file didn't pass the validation or an XMLHttpRequest error occured during the file upload |
success | file - Object - File objectresponseText - String - The XMLHttpRequest responseTextload - ProgressEvent - Native ProgressEvent event object | Emitted when the file processing and the XMLHttpRequest transaction has been completed successfully |
successmultiple | files - Array - Array of file objectsresponseText - String - The XMLHttpRequest responseTextload - ProgressEvent - Native ProgressEvent event object | Emitted when uploadMultiple is true and the file processing and the XMLHttpRequest transaction has been completed successfully |
removedfile | file - Object - File object | Emitted when a file was removed by the user |
reset | Emitted when all files have been removed by the use | |
error | error - String - Error message | Emitted when any error occured during the drag and drop or during getting the files from the file picker dialog |
maxfilesexceeded | file - Object - File object | Emitted when the maximum allowed number of files has been reached |
keydown | event - KeyboardEvent - Native keydown KeyboardEvent object | Emitted when the drop zone has focus and a key has been pressed |
focus | event - FocusEvent - Native FocusEvent object | Emitted when the drop zone gets focused |
blur | event - FocusEvent - Native FocusEvent object | Emitted when the drop zone has lost focus |
<wui-dropzone-file-list>
<wui-dropzone-file-list>
Component aliases<wui-dropzone-file-list>
Properties<wui-dropzone-file-list>
Slots
Component aliases
<wui-dropzone-file-list>
can also be used via the following aliases:
<wui-file-list>
Note: component aliases are only available when importing all of WuiVue or using the component group plugin.
Properties
Property | Type | Default | Description |
---|---|---|---|
tag | String | "UL" | The HTML tag for the file list |
Slots
Name | Scoped | Description |
---|---|---|
default | No | Content to place in the file list |
<wui-dropzone-file-list-item>
<wui-dropzone-file-list-item>
Component aliases<wui-dropzone-file-list-item>
Properties<wui-dropzone-file-list-item>
Slots<wui-dropzone-file-list-item>
Events
Component aliases
<wui-dropzone-file-list-item>
can also be used via the following aliases:
<wui-file-list-item>
Note: component aliases are only available when importing all of WuiVue or using the component group plugin.
Properties
Property | Type | Default | Description |
---|---|---|---|
addDownloadLink | Boolean | false | Whether or not a download action button will be displayed in the preview file list |
bytesSent | Number | 0 | The number of bytes which already have been sent |
compact | Boolean | false | If true, renders component in compact layout |
downloadUrl | String | "" | The url to download the file |
errors | Array | [] | An array of objects/errors in format [{ code: String, message: String }] |
fileName | String | required - The file name | |
id | String | required - The unique file id | |
imageUrl | String | "" | The thumbnail image url |
noRemoveLink | Boolean | false | Whether or not the remove icon button should be hidden |
progress | Number | 0 | The current processing/upload progress in percent |
size | Number | 0 | The file size in bytes |
status | String | required - The file status. Allowed values are added , queued , uploading , canceled , error and success | |
tag | String | "LI" | The HTML tag of the root element |
translations | Object | The translation object to pass custom texts | |
type | String | required - The file's mime type |
Slots
Name | Scoped | Description |
---|---|---|
actions | No | Content to place next to the download and remove icon buttons |
Events
Event | Arguments | Description |
---|---|---|
download | id - String - The file id | Emitted when user clicks on the download button |
remove | id - String - The file id | Emitted when user clicks on the remove button |
cancel-upload | id - String - The file id | Emitted when user clicks on the cancel upload button |
Importing individual components
You can import individual components into your project via the following named exports:
Component | Named Export |
---|---|
<wui-dropzone> | WuiDropzone |
<wui-dropzone-file-list> | WuiDropzoneFileList |
<wui-dropzone-file-list-item> | WuiDropzoneFileListItem |
Example
import { WuiDropzone } from "@wui/wui-vue/lib";
Vue.component("wui-dropzone", WuiDropzone);
Importing as a Vue.js plugin
This plugin includes all of the above listed individual components. Plugins also include any component aliases.
Named Export | Import Path |
---|---|
DropzonePlugin | @wui/wui-vue/lib |
Example
import { DropzonePlugin } from "@wui/wui-vue/lib";
Vue.use(DropzonePlugin);